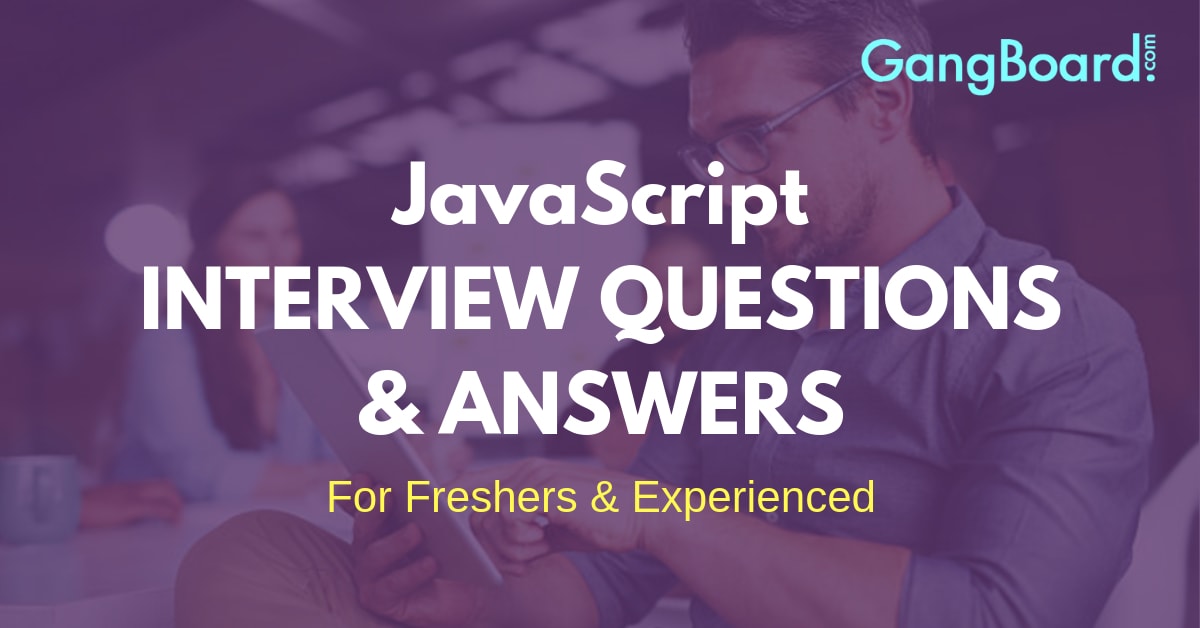
JavaScript Interview Questions and Answers
JavaScript Interview Questions and Answers
In case you’re searching for JavaScript Interview Questions and answers for Experienced or Freshers, you are at the correct place. GangBoard offers Advanced JavaScript Interview Questions and answers that assist you in splitting your JavaScript interview and procure dream vocation as JavaScript Developer. JavaScript is the highly desirable skill in IT and various other industries. The training on scripting language is given for developers and web designers. People wishing to get their career as developer, or a web designer are requested to go through an effective training with Javascript and prepare them with set of questions for any IT interview purposes. The questions provided by the centre have many practical knowledge-oriented questions, so that the candidate will have a real time learning experience while preparing for interviews. The concepts captured on these questions are from scratch and that is extended to advanced level of preparation. Though many institutes are providing the materials for interview preparation, the below listed questions sounds effective and elaborative.
Q1) What are all the data types JavaScript supports?
Answer: JavaScript offers seven data types which are: Number, String, Boolean, null, undefined, Symbol (newly added in ECMAScipt 6) and Object.
Q2) How can you remove an element from an array? Or can you use delete to remove an element in an array or not?
Answer: First, we should not use delete to remove an element from an array. Because, delete removes only the value of the array element, not the entire element. So the index still have undefined. And the length does not change. delete is supposed to use on an Object to remove its property.
The best way to remove an element is using splice method. The syntax is:
arr.splice(startIndex, deleteCount, itemToInser); and [1, 2, 3].splice(1, 1); result in [1, 3]. |
Q3) What are all the types of function? Or what are all the different ways you can declare a function and how they differ?
Answer: There are mainly three ways, we could define a function:
Function declaration: This is the straight-forward method. It uses a function keyword followed by the name of the function, list of parameters and a pair of curly braces to define function body. This type of definition is hoisted, means this block will be lifted up to the top of the scope and can be invoked before the declaration.
function isEven(num) { return num % 2 === 0; } |
var isEven = function(num) { return num % 2 === 0; }; |
(function(num) { return num % 2 === 0; })(2); Or, the below is also the same. (function(num) { return num % 2 === 0; }(2)); |
And finally we have Arrow functions and Generator functions which are newly added in ECMAScript 6.
Q4) What is instanceof in JavaScript?
Answer: This operator tests whether an object has the prototype property of a given constructor in its prototype chain. For example,
function Car(name) { this.name = name; } var mycar = new Car('Honda'); mycar instanceof Car; //true mycar instanceof Car; returns true since the mycar is constructed from the Car. |
Q5) How can you get the list of all properties in an Object?
Answer: The easy way is using Object.keys(). This will return an array of given object’s own enumerable properties.
If we want all properties, even not-enumerable properties also, we can use Object.getOwnPropertyNames().
Q6) What is Event capturing and Event bubbling?
Answer: It defines in which order the event handler should be executed. For example, consider an element which has click event attached, and its parent also has the same event handler attached to it. Now if the children has been clicked, then:
- In event capturing mode, the parent element’s event handler is executed first and then the child element’s event handler is executed after that.
- In event bubbling mode, the child element’s event handler is executed first and then the parent element’s event handler is executed after that.
Simply saying, the Event capturing is capturing the event handlers in top-down direction and Event bubbling is capturing the event handlers in bottom-top directions.
We can define which mode should be followed. In the EventListener syntax add the third parameter as true or false. The default value is false and the default execution phase is bubbling.
element.addEventListener(‘click’, doSomething, true) //Event handler is set to capturing phase.
element.addEventListener(‘click’, doSomething, false) //Event handler is set to bubbling phase.
Q7) What is the difference between window.onload and onDocumentReady?
Answer: onDocumentReady event is fired when DOM is loaded and it is safe to do any DOM manipulation after this event, so that the element is in the DOM. It will run prior to image and external contents are loaded.
window.onload will wait until the entire page is loaded including its CSS, images and fonts.
Q8) What is exception handling?
Answer: Exception handling is handling run time error in program. JavaScript provides try…catch statement to mark a block of code that can throw error in run time. If an exception is thrown at try block, the control will shifts to catch block. If no exception thrown, the catch block will be skipped. There is also one additional block, finally, at the end. It will run regardless of whether the try block failed or not.
Q9) What is shift() and push? How they are differing from each other?
Answer: ”The shift() removes the first element from an array and return it.’’ On the otherhand, unshift() adds the given element at the start of an array.” The push() adds the given element at the end of the array. “And, pop() removes the last element from an array.”
Q10) What is callback? How they work?
Answer: JavaScript allows a regular function to be passed as an argument to another function. This callback function will be invoked later inside the passed function. It is also called high-order functions. A common usecase for callback is event handlers.
$("#btn").click(clickEvent); |
Q11) What is Browser Object Model or BOM?
Answer: This is used to access the Browser window. Using the global object window, we can access the browser. They provide many useful methods like:
- console: accessing the console of the browser
- alert, window.confirm and window.prompt: showing alerts or getting input from the user.
- history, window.navigator: accessing history API and getting browser informations.
window.location: redirecting user or change urls
Q12) What is negative infinity?
Answer: The negative infinity is a number in JavaScript, which can derived by dividing a negative number by 0. Negative infinity is less than zero when compared, and positive infinity is greater than zero.
Q13) What is Number and String Objects in JavaScript?
Answer: JavaScript have Number and String objects to work with the primitive types number and string. We don’t need to explicitly create and use Number and String objects. JavaScript uses them to convert the primitive types to object when we are accessing any properties or methods on the primitive types. For example,
var str = 'Javascript'; str.length //10 |
var str = new String('Javascript');. The latter will return object. var str = new String('Javascript'); typeof str; //object |
Q14) What is encodeURI and decodeURI in JavaScript?
Answer: The encodeURI is used to encode an URL by replacing special characters, like space, percent. For example,
encodeURI(‘www.example.com/this is a test url with %character’) will results in, www.example.com/this%20is%20a%20test%20url%20with%20%25character. Notice that the space and percentage symbol are replaced with %20 and %25. The decodeURI works in reverse way.
Q15) What is currying in JavaScript?
Answer: Currying means constructing a function that process with partial parameters and return a function which when invoked would accept remaining parameters and return the final output.
A simple example is,
var greeting = function(greeting) { return function(name) { console.log(greeting + " " + name + "!"); }; }; When invoking it, var greetHello = greeting( "Hello" ); greetHello( "World" ); // Hello World! greeting( "Javascript" ); //Hello Javascript! We can also call the function immediately, greeting( "World" )( "World" ); // Hello World! |
Q16) What is NaN in Javascript?
Answer: NaN means Not-A-Number—the same as the value of Number.NaN. Several operations can lead to NaN result. Some of them are,
2 / 'a'; Math.sqrt(-2) Math.log(-1) 0/0 parseFloat('foo') The typeof NaN returns number. And comparing NaN with NaN yields false. NaN === NaN // false |
Q17) What is DOM
Answer: Document Object Model .
Q18) What is ES?
Answer: Ecma Script.
Q19) What is Javascript?
Answer: Scripting language, client side and server side both.
Q20) Is javascript case Sentiative?
Answer: yes.. variable and functions namespace.
Q21) Which is latest release in ECMAScript?
Answer: ES8.
Q22) Who is the founder of Javascript?
Answer: Netscape Navigator.
Q23) What is the difference between window and document?
Answer: window will be your current window of computer and document will be your current documenent in your page.
Q24) What are the difference between == and ===?
Answer: == checks only with data, but === checks data with datatype.
Q25) What is the use of typeof?
Answer: typeof will give the datatype of variable.
Q26) What is prototype in javascript?
Answer: Every object has prototype in javascript, so you can add the property to an object based on prototype. You can create the instant.
Q27) What is undefined?
Answer: In javascript, undefined means, variable is declared but doesn’t contain value.
Q28) What is not defined?
Answer: Varaible not yet declared.
Q29) What is closure?
Answer: variable have first its own scope, parent scope and global sope.
Q30) How do you empty an Array?
Answer: array.length = 0;
Q31) Check whether Object is an Array/Not?
Answer: isArray method.
Q32) What is instanceOf?
Answer: checks the current Object and Returns true, If the object of specified type.
Q33) How to find the length of associative Array?
Answer: Object.keys(counterarray).length
Q34) What is method?
Answer: It is a piece of code, associated with the object.
Q35) What is object?
Answer: It is collection of key value pair.
Q36) What is function?
Answer: It is a piece of code, but not associated with the object.
Q37) Syntax for Spread Attributes?
Answer: [ … {object} ].
Q38) What is the difference for let and const?
Answer: let will scope for block level and const values never change.
Q39) What is variable hoisting?
Answer: All the variable will go the top of the function and declare the variable.
Q40) What is IFFE?
Answer: Self executing Functions — no need to call the functions.
Q41) What is Default Parameters?
Answer: Sending the values as paramers in default context.
Q42) what is promise?
Answer: Used for asynchronous interations. it has 3 internal states, pending, fulfilled and rejected.
Q43) How to create an Object?
Answer: Object.Create();
Q44) What is function binding?
Answer: It is in advance javascript. Handles the events and callback functions in context of passing function in parameters.
Q45) What is constructor?
Answer: Constructor are defines with the fuctions. create a new object and pass value to this and returns new object.
Q46) Singleton Pattern in js?
Answer: It gives a way to code the logical unit, which can be accedes through single variable.
Q47) What are the data types are available in JavaScript (JS)?
Answers: Below is the list of data types which support in JS.
- Undefined
- Null
- Boolean
- String
- Symbol
- Number
- Object
48) How can you create an Array in JavaScript?
Answers: There are several ways to create array in JS.
var employeeDetails = [0]; employeeDetails [0]=” EMP”; var employeeDetails = [111,”JOHN”, “80K”]; var employeeDetails = new Array("EMP_ID", "EMP_NAME", "SALARY"); |
Q49) What are the ways to create an object in JavaScript?
Answers: Object concepts works in JS very well. Below are the ways to do the same.
- Object literals
- Using Functions
Object literals
<script> var empObj = { name : "", age : "", displayInfo : function() { document.write(this.name); document.write(this.age); } } |
Using Functions
<script> function f2(id, age) { this.id = id; this.age = age; this.displayInfo = function() { document.write(this.id); document.write(this.age); } } |
var obj = new f2("John", 24); </script> |
Q50) What is function in JS and how to define it?
Answers: Function used to be defined as a name following by function keyword, below is the example for the same.
function f5(){ // write code here }
Q51) What is Array helper function and explain one of them?
Answers: forEach, map,filter,find,every,some,reduce
forEach – It executes using the refer function for each element of the array, passing the array element as an argument. Below is the example for the same.
var fruits = ['Mango', 'Apple', 'Orange'] function print(val) { document.write(val) }fruits.forEach(print) |
Q52) What is every and find in array helper function?
Answers: Every – If each element of array passes the implementation of function, will be returned true or false.
Find – Finds the first element that passes the implementation of function, will be returned true or false.
Q53) What are the scopes of a variable in JavaScript?
Answers: JavaScript variable will have only two scopes.
- Global Variables – Variable which be defined or declare outside the function or visible for all known as global variable.
- Local Variables – Function parameters and variable defined inside function know as local variable.
Q54) What is every and some in array helper function?
Answers:
Every – If each element of array passes the implementation of function, will be returned true or false.
Some – It Checks if any element of the array passes implementation of defined function, which will returned true or false.
Q55) What is reduce array helper function and how to use it?
Answers: Reduce – This is used to reduce output to single value. It performs on implementation of function (accumulator) and will pass as first parameter and initial value of accumulator will be second parameter.
Below is the example for the same.
var array = [1, 2, 3, 4] function sum(acc, value) { return acc + value }var sumOfElements = array.reduce(sum, 0) document.write('Sum of', array, 'elements are', sumOfElements) |
Q56) What is Arrow functions and how to define it?
Answers: Simple functions requires lot of unnecessary code / boilerplate which can simplifies using arrow function.
var array = [1, 2, 3, 4] const sum = (acc, value) => acc + value var sumOfElements = array.reduce(sum, 0) document.write('Sum of', array, 'elements are', sumOfElements) |
Q57) What is Template strings in JS
Answers: This feature is introduce in ES6 , It makes easy String with placeholder of variable.
Below is the example for the same.
function display(firstName, lastName) { return `Good morning ${firstName} ${lastName}! What's up?` } document.write(display('Jan', 'Kowalski'))[/su_table]
Q58) How to give default argument in function?
Answers:
function print(arr = [], direction = 'A1`) { document.write('Array Printing', arr, direction) } print([1, 2, 3, 4]) print([1, 2, 3], 'A2') |
Array Printing [1,2,3,4] A1Array Printing [1,2,3,4] A2 |
Q59) How to merged two arrays?
Answers:
var array1 = [1,2,3] var array2 = [4,5,6,7] var mergedArrays = [...array1, ...array2] document.write('Merged arrays', mergedArrays) |
Q60) What is the use of ‘This’ operator in JavaScript?
Answers: This keyword refers to the object. Has to maintain different values depend on object.
Q61) What are the ways to define a variable in JavaScript?
Below are three ways to defined variable in JS
Var – This is used to declare a variable and can initialize the value of this variable. Before execution of code , variable declaration processed.
Const – const functions is not allow them to modify the object on which they are called, const variable used to define as constant value which will be common throughout the code.
Let – It’s allowed to reassign the value inside function but the value of same variable will be same which was outside function.
Q62) What is the difference between the operators ‘==‘ & ‘===‘?
Answers: The difference between “==” and “===” operator is works on type of variable and value. If number with a string and numeric literal allows to compare using == but === always give false as === check type of both variable then compare vale. So accordingly it will be giving true and false.
Q63) What is the difference between null & undefined?
Answers: undefined and null are two distinct types , null is an object and undefined is a type itself. Undefined means a variable has declared but not assigned any value. Null is an assignment value it can assign to any variable which can assign to variable that has to represent no value.
Q64) What is the difference between undefined and undeclared?
Answers: Undefined variables are those that are declared in the program but have not been given any value. Undeclared variables are those that do not exist in a program and are not declared.
Q65) What is the difference between document and window in JavaScript?
Answers: The document comes under the window and can be known or considered as a property of the window. Window is a global object which holds location , variables, functions, and history.
Q66) What is a prompt box in JavaScript?
Answers: A prompt box is a box which allows the user to pass the input in provided text area ,prompt displays dialog box which will be contain “OK” and “Cancel” to process further after entering the input value.
Q67) What is the use of isNaN function?
Answers: This works on number, this function will return true if the argument is not a number else it will give false.
Q68) How to give comments in Javascript?
Answers:
Single line comment - // use to give single line comments. Multiple line comments - /* Multi Line Comment */ |
Q69) Which are types of Pop up boxes available in JavaScript?
Answers:
- Alert
- Confirm
- Prompt
Q70) What would be the result of 1+2+”9″?
Answers: Here 1 and 2 integers, these two will be added and will be concatenated with 9 so the result would be 39.
Q71) What is the use of Void (0)?
Answers: Is used to call a method without refreshing the page
Q72) What is the data type of variables of in JavaScript?
Answers:
All variables in the JavaScript are object data types.
- Numbers
- String
- Boolean
- Null: This type has only one value: null.
- Undefined
- Object
Q73) What is the difference between a confirmation box and an alert box and?
Answers: Alert display only OK button but confirmation displays two OK and Cancel , which returns true and false.
Q74) Which keyword is used to print the text on the screen?
Answers: document.write(“Hi What’s up”) is used to print the text – Hi What’s up on screen.
Q75) Which operator supports in JavaScript?
Answers:
- Arithmetic Operators
- Comparison Operators
- Logical (or Relational) Operators
- Assignment Operators
- Conditional (or ternary) Operators
Q76) What will be the output for the below
document.write(0.1 + 0.2);
document.write(0.1 + 0.2 == 0.3);
Answers:
0.30000000000000004
false
Q77) How to read elements of an array in JavaScript?
Answers:
var num1 = [1, 2, 3, 4, 5]; for (var i = 0; i < num1.length; i++) { document.write(num1[i]); } |
Q78) What is callback in JS?
Answers: Callaback is a plain JavaScript function passed to some method as argument or option. Callback can make events like if any state is matched then can trigger to some other function.
Q79) What is closure in JS?
Answers: Whenever a variable is defined outside the current scope is accessed from within some inner scope closure will be created.
Q80) Which built-in method returns the length of the string?
Answers: String length can get using length() method.
Q81) What is Difference between the substr() and substring() functions in JavaScript?
Answers:
It’s a function work in this form substr(start_Index, length).
It returns the substring from start_Index and the returns ‘length’ number of characters.
var s1 = "hello"; ( s1.substr(1,4) == "ello" ) // true
Q82) What is spread operator in JavaScript?
Answers: The spread operator works on expression in places where multiple argument/variables/elements are needed to be present and It represents with three dots (…). Below is the example for the same.
For example:
var mid = [3, 4];
var newarray = [1, 2, …mid, 5, 6];
document.write(newarray);
// [1, 2, 3, 4, 5, 6]
Q83) What is Promise in JavaScript?
Answers: It’s is a object which produce a value that might be give result in the future. The value can be resolved value or it can be a reason as well which tells why the value is not resolved.
A promise has 3 states.
Fulfilled – Operation completed and the promise has a specific value.
Rejected- Operation failed and It has a reason to shows why this operation failed.
Pending- The operation say’s it’s pending not in reject or fulfilled.
Q84) What is “use strict”?
Answers: This has included in ES5. This directive enforces the code to be executed in strict mode. For an example strict mode will not allow to use a variable without declaring it.
Q85) How to create blink text using JS ?
Answers:
<html> <body> <script> var str1 = "Life is all about the next step"; var response = str1.blink(); document.write(response) </script> </body> </html> |
Q86) What are different types of errors available in JS?
Answers:
Load time error – This error come due to syntax error or can be a reason if allocate resource not available.
Run time error – This Error comes can come due to command inside the HTML or any un expected conditional scenario performed.
Logical Error – This error comes due to break of Business logic which code has written inside function or outside function.
Q87) What close() does in JavaScript
Answers: This method is used to close the current window. Must write window.close() to ensure that this command is associated with a window object.
Q88) Is exception handling possible ? How to handle exception in JavaScript ?
Answers: Exception handling is possible; and this can be achieved using the following keywords try, catch and finally.
Try { //BL Logic } Catch(arrayindexoutofboundsexception a1) { //This will catching ArrayIndexOutBoundException } |
Q89) How is it possible to get the total number of arguments that are passed to a function?
Answers: The arguments.length property helps to getting the total number of arguments that are passed to function.
Q90) What is the difference between instanceof and typeof in Javascript?
Answers: The typeof operator returns a string of what type the operand is. instanceof operator works with objects and checks on what type the object is.
Q91) What is BOM ?
Answers: It stands for Browser Object Model. BOM is using for interaction with a browser is possible. Default object of the browser is a window.
Q92) What is Classes and how to define?
Answers: ES6 classes are a simple and prototype-based OO pattern. Classes contain methods and support prototype-based inheritance, instance and static methods and constructors.
Below is the snippet code for the same.
class VehicleAndParts { constructor(make, model, color) { this.make = make; this.model = model; this.color = color; } getName() { return this.make + " " + this.model; } } |
Q93) What can be output for this JS code?
var x1 = 1; var output = (function(){ delete x1; return x1; })();document.write(output);
Answers:
The output would be 1. The delete used to perform to delete the property of an object. Here x1 is not an object, but it’s a global variable.
Q94) What will be the output of the code below?
var x1 = { foo : 1}; var output = (function(){ delete x1.foo; return x1.foo; })(); document.write(output); |
foo is a self invoking function, we should delete the “foo” property from object.The output will be undefined,
Q95) What will be the output of the code below?
var Employee = { company: 'CompanyBQ' } var emp2 = Object.create(Employee); delete emp2.company document.write(emp2.company); |
The output would be CompanyBQ. Here, emp2 object has company as its prototype property. The delete operator will not delete prototype property.
Q96) When is the use JSON.stringify()?
We use this method to convert a JavaScript Json data to a string.
Q97) What is “With ” keyword in JavaScript?
Answers: object’s properties or methods, use the with keyword.
The syntax:
with (obj){ properties used without the object name and dot } |