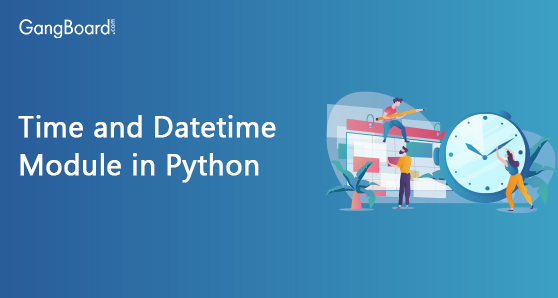
Time and Datetime Module in Python
Time and Datetime Module in Python
Python has an inbuilt module to deal with date and time. Date and time are very essential in any project to record the real-time data.
Time Module:
Python time module is helpful when we need the current system time into our code. we may need to synchronize the system time with our project. localtime() is the function to get the system time.
# import the time module import time seconds = time.time() Now = time.localtime(seconds) # print the currentTime variable to know about it print(Now,’\n’) print(‘The Current System time is :’, time.asctime(Now)) We can format the time using strftime() to format the time. print(‘Time is :’, time.strftime(“%d/%m/%Y”, Now)) |
Calendar Module:
Python has an inbuilt module to check the current date and month using the Calendar module.
# import Calendar import calendar #current month print(‘The month August is:\n’, calendar.month(2020, 8)) # we can set the first day of the week as sunday calendar.setfirstweekday(6) # print the calender print(‘The month August is:\n’, calendar.month(2020, 8)) # Checking year is leap year print(‘2020 a leap year? Ans:’, calendar.isleap(2020)) print(‘1998 a leap year? Ans:’, calendar.isleap(1998)) |
datetime Module:
Let us know about datetime module in python. Using datetime it is easy to print the current date and time.
import time import datetime print(“unix epoch started: %s”, time.time()) print(“Present date and time: ” , datetime.datetime.now()) We can do lot of thing using datetime module. We can print the current date, time, year, month etc.. import datetime print(“Current year: “, datetime.date.today().strftime(“%Y”)) print(“Current Month of year: “, datetime.date.today().strftime(“%B”)) print(“Week number of the year: “, datetime.date.today().strftime(“%W”)) print(“Weekday of the week: “, datetime.date.today().strftime(“%w”)) print(“Day of year: “, datetime.date.today().strftime(“%j”)) print(“Day of the month : “, datetime.date.today().strftime(“%d”)) print(“Day of week: “, datetime.date.today().strftime(“%A”)) |
import datetime #Getting week day for a date My_birthday = datetime.date(1989,10, 1) #year, month, day print(My_birthday.strftime(“%A”)) |
Getting current date:
import datetime today = datetime.date.today() print(today) |
Get date from a timestamp:
We can get the date from timestamp also. The time stamp is usually the number of seconds between the particular date and January 1,1970 at UTC. We can convert the time stamp using the function fromtimestamp().
from datetime import date timestamp_now = date.fromtimestamp(1326244364) print(“Date =”, timestamp_now) |
Get today’s year, month and day:
We can print year, month and day using datetime module
from datetime import date today = date.today() print(“Present year:”, today.year) print(“Present month:”, today.month) print(“Present day:”, today.day) |
datetime.datetime:
The datetime module has a class named dateclass that can contain information from both date and time objects.
from datetime import datetime #datetime(year, month, day) a = datetime(2020, 10, 18) print(a) # datetime(year, month, day, hour, minute, second, microsecond) b = datetime(2020, 11, 28, 23, 55, 59, 342380) print(b) |
datetime.timedelta:
timedelta function is very useful while we want to calculate the future datetime and past datetime.
from datetime importdatetime,timedelta Today=datetime.now() # what is the date after 45 days from today future=Today+timedelta(45) print(future) # what is the date before 67 days from today past=Today-timedelta(67) print(past) |
Difference between two timedeltas:
from datetime import timedelta time1 = timedelta(weeks = 2, days = 5, hours = 1, seconds = 33) time2 = timedelta(days = 4, hours = 11, minutes = 4, seconds = 54) time3 = time1 – time2 print(“t3 =”, time3) |
Dealing with TimeZones:
In Realtime we have to work on different clients situated in different time zones. we can handle all time zones in python using pytz module. Pytz is third party library. We have to install it using pip install pytz.
from datetime import datetime importpytz #local time local = datetime.now() print(“Local:”, local.strftime(“%m/%d/%Y, %H:%M:%S”)) #newyork time zone tz_NY = pytz.timezone(‘America/New_York’) datetime_NY = datetime.now(tz_NY) print(“NY:”, datetime_NY.strftime(“%m/%d/%Y, %H:%M:%S”)) #Europe time zone tz_London = pytz.timezone(‘Europe/London’) datetime_London = datetime.now(tz_London) print(“London:”, datetime_London.strftime(“%m/%d/%Y, %H:%M:%S”)) |
Print all time zones:
To get all time zones. The list is quite big. We will be using some 4 or 5 time zones in our real-time projects.The below program will display all time zones.
importpytz #Displays all time zones print(‘all_timezones =’, pytz.all_timezones, ‘\n’) |
Python pytz example:
importpytz from datetime import datetime # getting utctimezone utc = pytz.utc # getting timezone by name ist = pytz.timezone(‘Asia/Kolkata’) # getting datetime of specified timezone print(‘UTC Time =’, datetime.now(tz=utc)) print(‘IST Time =’, datetime.now(tz=ist)) |