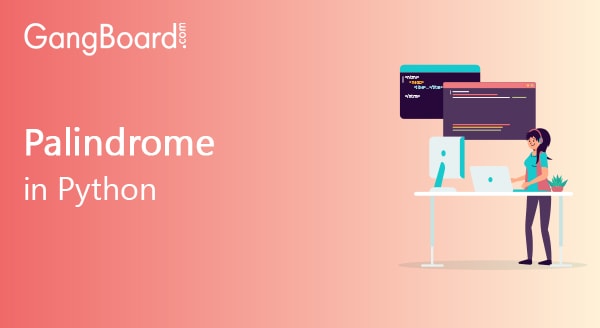
Palindrome in Python
Palindrome in Python
In general, Palindrome is a sequence of alphabets or numbers that appears the same when read from the start to end or from the end to start. Some examples are
- Mom
- Dad
- Civic
- Radar
In the case of a series of numbers, a number is said to be a palindrome number if the complete reverse of the number is still numerically equal to the original number before reversing.
- 1005001
- 9005009
- 8990998
- 7800087
Now we will see how a simple Python program can be written to verify if a number is a palindrome or a non-palindrome.
Objective:
The goal is to check if the input number is a palindrome number or not and print the respective result.
Method of verification
- Get an input number from the user by the means of the input() method.
- The first step is to verify whether the number obtained as input is an integer or not.
- In order to make the overall operation easy, the number present in integer format is converted to string pattern by the usage of str() method.
- Now we employ a slightly advanced technique whereby we chuck the starting and empty places and perform an operation to reverse the string.
- This is the step of evaluation whereby we have to compare the original number and the reversed one to see if they are the same as each other.
- If both the numbers match with each other, we can conclude that the number given is a palindrome.
- Else if the case is not as such, then the most obvious inference is that the number is non-palindrome.
number = input (‘Please enter a number to check: ‘) try : value = int (number) if number == str (number) [ :: -1 ] : print (‘The input number is a Palindrome’) else: print (‘The input number is not a Palindrome’) except ValueError: print (‘The input number is not valid’) |
Output
INPUT: 10901 OUTPUT: The input number is a Palindrome INPUT: 898989 OUTPUT: The input number is not a Palindrome INPUT : -9939AGDJ OUTPUT: The input number is not valid |
Now that you know to check if a number is a palindrome or not, the next step is to start learning to write a Python program to check whether an input string is a palindrome or not.
Objective – The goal is to check if the input string is a palindrome or not and print the respective result.
Method of verification
- Get an input string from the user by the means of the input() method.
- Initiate a variable and store the input string in it.
- Reverse the obtained string by the means of string slicing and compare it to the original string.
- In the case where both the strings match, then the input string can be declared as a palindrome.
- In the other case where the strings do not match, then it can be declared that the input string is not a palindrome
Consider the following programming code
value = input (“Enter an input “) if (value == value [::-1]): print (“Input value is palindrome”) else: print(“Input is not palindrome”) |
Output
INPUT: sassas OUTPUT: Input value is palindrome INPUT: idsudus OUTPUT: Input value is not palindrome |
Now we have seen how to write Python programs to check if a number or a string is Palindrome or not. Going further let us see how a loop can be employed to perform the same checking.
Consider the following program example:
string = input (“Enter a string value: “) val = “” for m in string: val = m + val if (string == val): print (“Input string is Palindrome: ”) else: print (“Input string is not Palindrome: “) |
Output
INPUT : poiop OUTPUT : Input string is palindrome INPUT: iowqi OUTPUT: Input string is not palindrome |
Now let us look at another alternate method for Palindrome number verification by a different logic.
Consider the below code sample:
inp = int (input (“Please enter number: “) temp = inp inp1 = 0 while ( int > 0 ) d = inp % 10 inp1 = inp1 * 10 + d inp = inp / 10 if (temp == inp1) print (“Input number is palindrome”) else: print (“Input number is not palindrome”) |
Output
INPUT : 10901 OUTPUT : Input number is palindrome INPUT : 898989 OUTPUT: Input number is not palindrome |
Finally, there is one another method for palindrome verification in which one can extract every single letter from the string and store it in a variable that is empty. The final step is to compare both the strings once all the characters from the original string are stored in the new string. Consider the following code logic:
str = “Python” x = “” for i in str x = i + x if (x == str) print (“Input string is Palindrome”) |
I hope this article helped you in advancing knowledge about Palindromes in Python programming language.